- Teacher: Eugen Richard Ardelean
- Teacher: Feier Mihai
- Teacher: Andrei Moldovan
- Teacher: Vlad-Andrei Negru
- Teacher: Eliza Maria Olariu
- Teacher: Remus Mircea Petrache
- Teacher: Raluca Portase
- Teacher: Vasile Suciu
- Teacher: Raluca Bozdog
- Teacher: Iulia Costin
- Teacher: Dan Domnita
- Teacher: Horatiu Florea
- Teacher: Ion Giosan
- Teacher: Vlad-Andrei Negru
- Teacher: Dan Toderici
- Teacher: Robert Varga
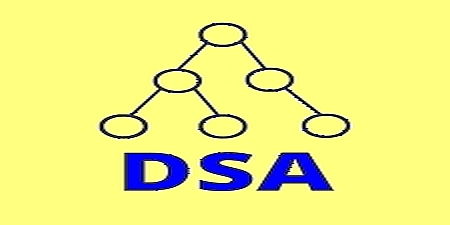
About the course (objectives, outline, recommended reading). Problem solving. Notions of Algorithmics (growth of functions, efficiency, programming model). Stacks, queues. Lists |
Trees – definitions, traversals. ADT Tree. Implementations. Binary Search Trees. |
Sets ADTs and Implementations. Dictionary ADT. Hash Tables. Mapping ADT. |
Priority Queue ADT. Tries |
Advanced Set Representation Methods. AVL trees. 2-3 Trees. Union-Find Set ADT. |
Directed Graphs. Definitions. Representations. ADT’s. Single Source Shortest Path Problem (Dijkstra, Bellman-Ford, Floyd-Warshall). Traversals for DGs. Parenthesis Lemma. DAGs. Topological Sort |
Undirected Graphs. Terminology. Free Trees. Graph Representations. Graph Traversals (depth-first, breadth-first). Articulation points & Biconnected Components. |
Algorithm Design Techniques I. Brute Force Algorithms. Greedy Algorithms. |
Algorithm Design Techniques I. Divide-and-Conquer. |
Algorithm Design Techniques II. Dynamic Programming. |
Algorithm Design Techniques III. Backtracking. Search Tree Strategies (branch and bound) |
Algorithm Design Techniques IV. Search Tree Strategies (branch and bound). Local Search. |
Sorting |
- Teacher: Pocol Ciprian
- Teacher: Marius Joldoș
- Teacher: Vlad Olteanu
- Teacher: Carla Elena Staicu
- Teacher: Dragos Varvara
- Teacher: Alexandru Viorel Bondor
- Teacher: Raluca Brehar
- Teacher: Victoria Cozma
- Teacher: Radu Drăgan
- Teacher: Andrei - Florin Hulea
- Teacher: Marius - Horatiu Totoian
- Teacher: Eugen Richard Ardelean
- Teacher: Paul Helmer
- Teacher: Rares-Liviu Horge
- Teacher: Anca Iordan
- Teacher: Camelia Lemnaru
- Teacher: Feier Mihai
- Teacher: Vlad-Andrei Negru
- Teacher: Eliza Maria Olariu
- Teacher: Ciprian Oprișa
- Teacher: Remus Mircea Petrache
- Teacher: Raluca Portase
- Teacher: Rodica Potolea
- Teacher: Vasile Suciu
- Teacher: Adrian Tirea
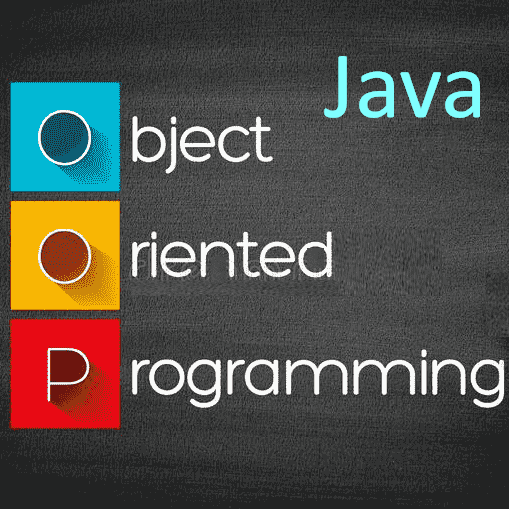
Concepts and paradigms in OOP. On to Java |
Control structures in Java. |
Classes and Objects. Arrays |
Packages. Inheritance and polymorphism. |
Java Interfaces. OO Application Development |
UML Object and Class Diagrams. Assertions. |
Testing. Debugging. Java Errors and Exceptions |
Java Collections. Generic Programming. |
Introduction to Java I/O |
Event handling in Java. Introduction to Java Graphics |
Graphical User Interfaces (I) |
Introduction to Threads |
Graphical User Interfaces (II) |
Review |
- Teacher: Bogdan Bindea
- Teacher: Alexandru Ștefan Garleanu
- Teacher: Alexandru-Ionuț Ivan
- Teacher: Marius Joldoș
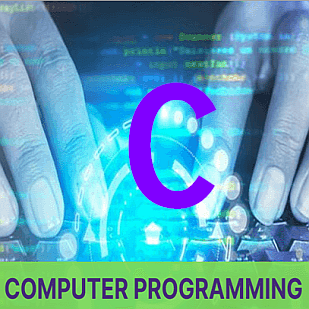
Programming Languages. Stages of Problem solving Using Computers. Algorithm – Definition, Properties. C features. Simple Data Types. Simple I/O |
Programming Style. Digital Representations. Variables and Expressions |
C Statements. C Preprocessing |
Functions (Structure, Invocation, Parameter passing, Functions as parameters, Variable scope). Functions for character processing |
Modular Programming. Debugging |
Pointers. Memory Management. |
Pointers and Arrays. Function Pointers |
C Character Strings. C library |
Structures, unions, enumerations. User-defined Types |
File Handling. High Level I/O. |
Recursion. Mechanism and Examples |
Working with time. I/O redirection. Variable length argument lists. Command line arguments. Self referential structures |
Sample Programs Explained. (Combinatorial generation. Simple Sorting Algorithms) |
Review |
- Teacher: Bianca - Veronica Avram
- Teacher: Pocol Ciprian
- Teacher: Radu Drăgan
- Teacher: Marius Joldoș
- Teacher: Vlad Olteanu
- Teacher: Dragos Varvara